今天在使用SpringAop的时候,在代码运行过程中,突然发生了异常,作者仔细检查了代码,最终发现,是因为SpringBoot所依赖的版本不同,导致SpringAop的执行顺序发生了变化导致的。
低版本SpringBoot Aop测试
SpringBoot 2.0.4.RELEASE版本依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.4.RELEASE</version> <relativePath/> </parent>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> </dependency> </dependencies>
|
Controller层接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @RestController @RequestMapping("user") public class UserController {
@GetMapping public String user() { System.out.println("[Controller]执行controller方法"); return "正常执行"; }
@GetMapping("e") public String e() { System.out.println("[Controller]执行controller方法:异常之前"); int i = 1 / 0; System.out.println("[Controller]执行controller方法:异常之后"); return "发生异常"; } }
|
AOP代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| @Aspect @Component public class LogAop {
@Pointcut("execution(public * com.hrp.controller.*.*(..))") public void logAop() { }
@Before("logAop()") public void doBefore() { System.out.println("[AOP]===================doBefore==================="); }
@AfterReturning("logAop()") public void doAfterReturning(JoinPoint joinPoint) { System.out.println("[AOP]===================doAfterReturning==================="); }
@AfterThrowing(value = "logAop()", throwing = "e") public void doAfterThrowing(JoinPoint joinPoint, Exception e) { System.out.println("[AOP]===================doAfterThrowing==================="); }
@After("logAop()") public void doAfter() { System.out.println("[AOP]===================doAfter==================="); }
@Around("logAop()") public Object doAround(ProceedingJoinPoint proceedingJoinPoint) throws Throwable { System.out.println("[AOP]===================doAround before==================="); Object result = proceedingJoinPoint.proceed(); System.out.println("[AOP]===================doAround after==================="); return result; } }
|
AOP执行结果
1 2 3 4 5 6
| [AOP]===================doAround before=================== [AOP]===================doBefore=================== [Controller]执行controller方法 [AOP]===================doAround after=================== [AOP]===================doAfter=================== [AOP]===================doAfterReturning===================
|
1 2 3 4 5
| [AOP]===================doAround before=================== [AOP]===================doBefore=================== [Controller]执行controller方法:异常之前 [AOP]===================doAfter=================== [AOP]===================doAfterThrowing===================
|
更换高版本的SpringBoot依赖
SpringBoot 2.3.2.RELEASE版本依赖
1 2 3 4 5 6
| <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.2.RELEASE</version> <relativePath/> </parent>
|
AOP执行结果
1 2 3 4 5 6
| [AOP]===================doAround before=================== [AOP]===================doBefore=================== [Controller]执行controller方法 [AOP]===================doAfterReturning=================== [AOP]===================doAfter=================== [AOP]===================doAround after===================
|
1 2 3 4 5
| [AOP]===================doAround before=================== [AOP]===================doBefore=================== [Controller]执行controller方法:异常之前 [AOP]===================doAfterThrowing=================== [AOP]===================doAfter===================
|
测试结果
- SpringBoot 2.0.4.RELEASE版本
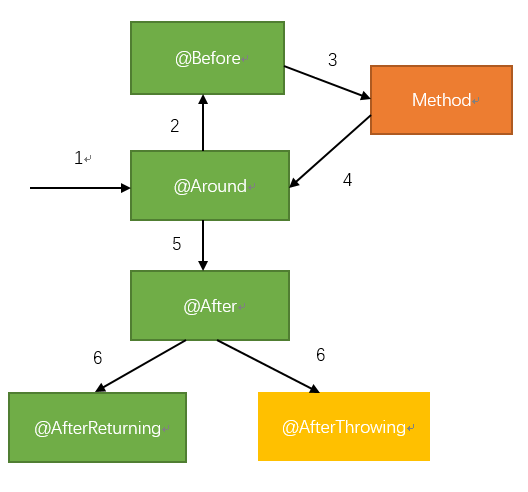
- SpringBoot 2.3.2.RELEASE版本
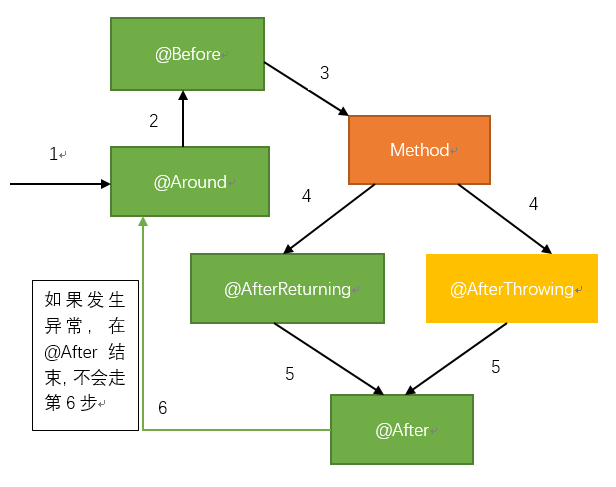