Spring-AMQP
什么是Spring-AMQP?
Spring-AMQP官网:http://spring.io/projects/spring-amqp
- Spring-AMQP是Spring的一个子项目,是对
amqp协议
的抽象实现。
什么是SpringBoot-AMQP?
我们先来看一下SpringBoot-AMQP的依赖。(不知道为什么,官网打开太慢了,截不了图,直接看依赖吧)
1 2 3 4
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency>
|
SpringBoot-AMQP是一个SpringBoot项目,可以直接使用SpringBoot为我们提供的启动器,快速的搭建一个Spring-AMQP的环境。
我们来看一下spring-boot-starter-amqp
都依赖了哪些依赖。
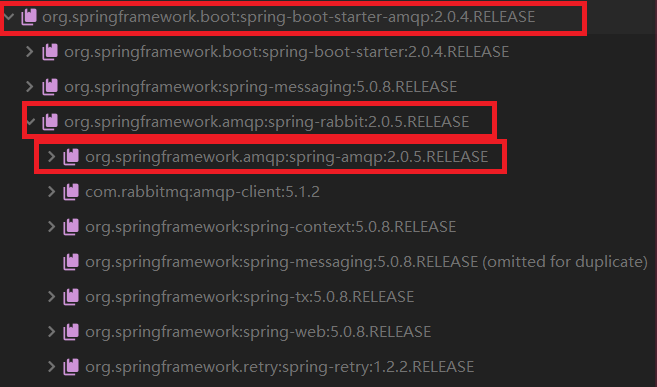
从上图我们可以看到,spring-boot-starter-amqp
是SpringBoot为我们封装的一个启动器,而spring-amqp
则是Spring对amqp协议的抽象实现,而spring-rabbit
则是具体实现,使用的正是RabbitMQ。
Spring-AMQP、Spring-Rabbit、SpringBoot-AMQP
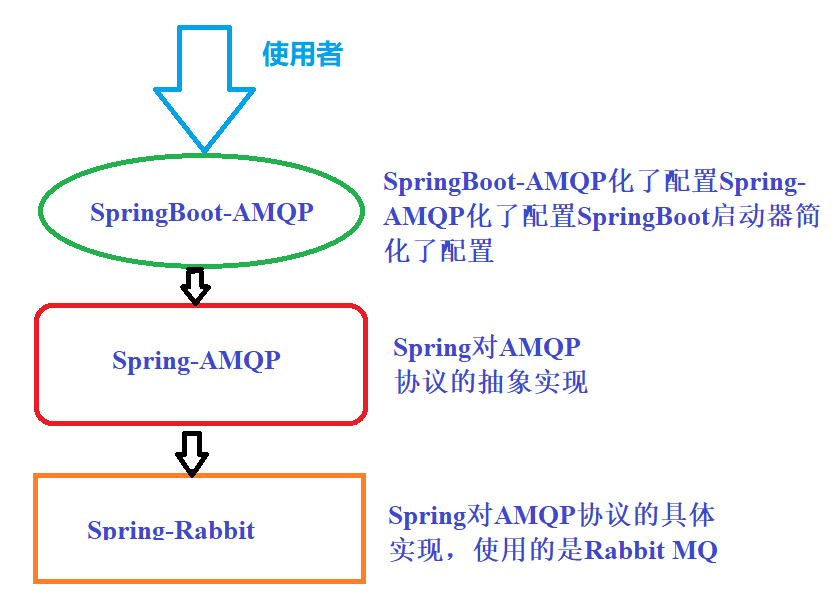
SpringBoot-AMQP
搭建项目环境
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.4.RELEASE</version> </parent>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
|
SpringBoot项目启动类
1 2 3 4 5 6
| @SpringBootApplication public class AmqpApplication { public static void main(String[] args) { SpringApplication.run(AmqpApplication.class); } }
|
application.yml配置文件
1 2 3 4 5 6 7
| spring: rabbitmq: host: 192.168.177.128 port: 5672 virtual-host: /mymq username: admin password: admin
|
创建监听者(消费者)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @Component public class Listener {
@RabbitListener(bindings = @QueueBinding( value = @Queue(value = "topic_exchange_queue_1", durable = "false"), exchange = @Exchange( value = "amq.topic", ignoreDeclarationExceptions = "true", durable = "true", type = ExchangeTypes.TOPIC ), key = {"insert"})) public void listen(String message){ System.out.println("接收到消息:" + message); } }
|
创建生产者
- 在这里,我们使用测试类代替生产者,在真正的项目环境中,生产者应该也是一个被Spring管理的类中的方法。
- AmqpTemplate类中有很多重载方法,可以视具体情况而选择具体方法。
1 2 3 4 5 6 7 8 9 10 11 12 13
| @SpringBootTest @RunWith(SpringRunner.class) public class AmqpTest {
@Autowired private AmqpTemplate amqpTemplate;
@Test public void test() { String message = "Hello World!"; amqpTemplate.convertAndSend("amq.topic","insert",message); } }
|